PsychoPy & Behaviour experiment I
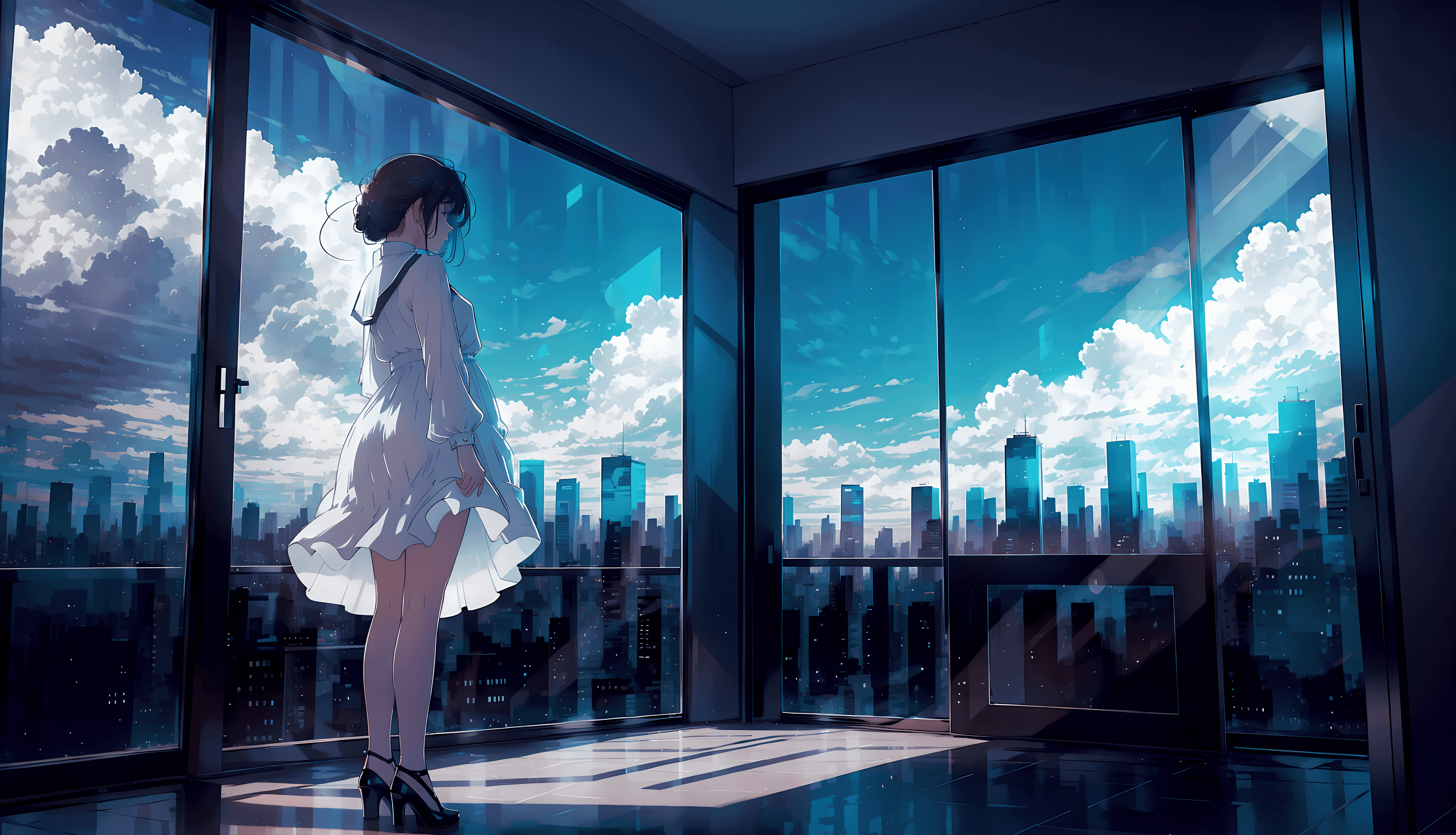
PsychoPy & Behaviour experiment I
ChaoIn behavioural science, particularly psychology and neuroscience research, conducting experiments is crucial for verifying hypotheses. Two decades ago, executing such experiments necessitated engineering and electrical skills to operate devices that could present image or sound stimuli. Researchers used stopwatches or other precise timing tools to collect data. Fortunately, with technological advancements, researchers no longer need to retreat to garages to test cumbersome equipment and draw far-fetched conclusions. Gone are the days of stopwatch-wielding researchers; now, it’s all about elegant screen clicks proving the Stroop Effect, perhaps while leisurely sipping coffee.
As noted in the preface of Building Experiments in PsychoPy, modern programming languages like Python and JavaScript enable the design of studies without requiring a Ph.D. in computer science. PsychoPy stands out as the most popular software in behaviour research. It offers a Graphic User Interface (GUI) for the ‘out-of-the-box’ enthusiasts and a command line for those who enjoy ‘reading binary for fun.’
While the GUI allows researchers an intuitive setup for ‘equipment’ (presenting stimuli and collecting response times), it arguably lacks the flexibility to fully customize the entire experiment’s process. We must admit that constructing experiments with mouse clicks and drags can appear as if one is a novice in an introductory computer science course, unfamiliar with coding. However, as a professional scientist, your realm should be coding in the terminal amidst a sea of black and green text. Therefore, I am crafting a post for my blog to assist beginners in learning how to use code to develop their Stroop experiments.
0x00 IMPORT MODULES & PATH SETTINGS
1 | # ===================== |
First, import the OS package to manage experiment files. Use the Time and Datetime packages, akin to the traditional stopwatch, for measuring response times in behavioural studies. The PsychoPy package, central to your study, allows selective function imports based on your research needs, avoiding the slowdown associated with loading the entire package. Utilize Random and Itertools for random stimulus presentation. For data formatting and analysis, Pandas and Numpy are your go-to tools. These packages are optional; you might not need them all depending on your study.
1 | # ===================== |
0x01 COLLECT PARTICIPANT INFO
1 | # ===================== |